Chat
Configure, send and intercept chat messages.
🗿Static Class
This is a Static Class. Access it's methods directly with
.
. It's not possible to spawn new instances.Examples​
Server/Index.lua
-- sends a chat message to everyone
Chat.BroadcastMessage("Welcome to the server!")
Static Functions​
Returns | Name | Description | |
---|---|---|---|
AddMessage | Adds a chat message which will display local only | ||
SendMessage | Sends a chat message to a Player only | ||
SetConfiguration | Configures the Chat visuals and position | ||
SetVisibility | Sets if the Chat is visible or not | ||
Clear | Clears all messages | ||
BroadcastMessage | Sends a chat message to all Players |

AddMessage
​
Adds a chat message which will display local only
Chat.AddMessage(message)
Type | Parameter | Default | Description |
---|---|---|---|
string | message |

SendMessage
​
Sends a chat message to a Player only
Chat.SendMessage(player, message)
Type | Parameter | Default | Description |
---|---|---|---|
Player | player | The player to receive the message | |
string | message | The message |

SetConfiguration
​
Configures the Chat visuals and position
Chat.SetConfiguration(screen_location?, size?, anchors_min?, anchors_max?, alignment?, justify?, show_scrollbar?)
Type | Parameter | Default | Description |
---|---|---|---|
Vector2D | screen_location? | Vector(-25, 0) | |
Vector2D | size? | Vector(600, 250) | |
Vector2D | anchors_min? | Vector(1, 0.5) | |
Vector2D | anchors_max? | Vector(1, 0.5) | |
Vector2D | alignment? | Vector(1, 0.5) | |
boolean | justify? | true | |
boolean | show_scrollbar? | true |

SetVisibility
​
Sets if the Chat is visible or not
Chat.SetVisibility(is_visible)
Type | Parameter | Default | Description |
---|---|---|---|
boolean | is_visible |

Clear
​
Clears all messages
Chat.Clear()

BroadcastMessage
​
Sends a chat message to all Players
Chat.BroadcastMessage(message)
Type | Parameter | Default | Description |
---|---|---|---|
string | message | The message to send to all Players |
Events​
Name | Description | |
---|---|---|
![]() | PlayerSubmit | Called when a player submits a message in the chat |
ChatEntry | Called when a new Chat Message is received, this is also triggered when new messages are sent programatically | |
Open | When player opens the Chat | |
Close | When player closes the Chat |
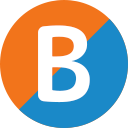
PlayerSubmit
​
Called when a player submits a message in the chat
Return false to prevent the message from being sent
Chat.Subscribe("PlayerSubmit", function(message, player)
-- PlayerSubmit was called
end)
Type | Argument | Description |
---|---|---|
string | message | The message sent by the player |
Player | player | The player who sent the message - on client it will always be the localplayer |

ChatEntry
​
Called when a new Chat Message is received, this is also triggered when new messages are sent programatically
This is useful for creating your own Chat interface while still use the built-in system
Chat.Subscribe("ChatEntry", function(message, player)
-- ChatEntry was called
end)
Type | Argument | Description |
---|---|---|
string | message | The message |
Player or nil | player | The player who sent the message or nil if this was called on client side or was sent through code |

Open
​
When player opens the Chat
Chat.Subscribe("Open", function()
-- Open was called
end)

Close
​
When player closes the Chat
Chat.Subscribe("Close", function()
-- Close was called
end)