Base Damageable
Base class for all Damageable entities. It provides Health and Damage related methods and events.
info
This is a base class. You cannot spawn it directly.
Functions
Returns | Name | Description | |
---|---|---|---|
integer | ApplyDamage | Do damage to this entity | |
![]() | integer | GetHealth | Gets the current health |
![]() | integer | GetMaxHealth | Gets the Max Health |
Respawn | Respawns the Entity, fullying it's Health and moving it to it's Initial Location | ||
SetHealth | Sets the Health of this Entity | ||
SetMaxHealth | Sets the MaxHealth of this Entity |

ApplyDamage
Do damage to this entity, will trigger all related events and apply modified damage based on bone. Also will apply impulse if it's a heavy explosion
— Returns integer (the damage applied).
local ret = my_damageable:ApplyDamage(damage, bone_name?, damage_type?, from_direction?, instigator?, causer?)
Type | Parameter | Default | Description |
---|---|---|---|
integer | damage | ||
string | bone_name? |
| |
DamageType | damage_type? | DamageType.Shot | |
Vector | from_direction? | Vector(0, 0, 0) | |
Player | instigator? | nil | The player which caused the damage |
any | causer? | nil | The object which caused the damage |
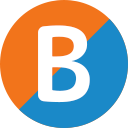
GetHealth
Gets the current health
— Returns integer.
local ret = my_damageable:GetHealth()
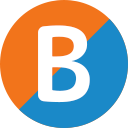
GetMaxHealth
Gets the Max Health
— Returns integer.
local ret = my_damageable:GetMaxHealth()

Respawn
Respawns the Entity, fullying it's Health and moving it to it's Initial Location
my_damageable:Respawn(location?, rotation?)
Type | Parameter | Default | Description |
---|---|---|---|
Vector | location? | initial location | If not passed will use the initial location passed when the Entity spawned |
Rotator | rotation? | Rotator(0, 0, 0) |

SetHealth
Sets the Health of this Entity. You can only call it on alive Entities (call Respawn first)
my_damageable:SetHealth(new_health)
Type | Parameter | Default | Description |
---|---|---|---|
integer | new_health |

SetMaxHealth
Sets the MaxHealth of this Entity
my_damageable:SetMaxHealth(new_max_health)
Type | Parameter | Default | Description |
---|---|---|---|
integer | new_max_health |
Events
Name | Description | |
---|---|---|
![]() | HealthChange | When Entity has it's Health changed, or because took damage or manually set through scripting or respawning |
![]() | Respawn | When Entity Respawns |
![]() | Death | When Entity Dies |
![]() | TakeDamage | Triggered when this Entity takes damage |
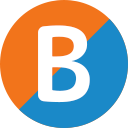
HealthChange
When Entity has it's Health changed, or because took damage or manually set through scripting or respawning
Damageable.Subscribe("HealthChange", function(self, old_health, new_health)
-- HealthChange was called
end)
Type | Argument | Description |
---|---|---|
Damageable | self | |
integer | old_health | |
integer | new_health |
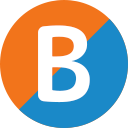
Respawn
When Entity Respawns
Damageable.Subscribe("Respawn", function(self)
-- Respawn was called
end)
Type | Argument | Description |
---|---|---|
Damageable | self |
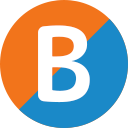
Death
When Entity Dies
Damageable.Subscribe("Death", function(self, last_damage_taken, last_bone_damaged, damage_type_reason, hit_from_direction, instigator, causer)
-- Death was called
end)
Type | Argument | Description |
---|---|---|
Damageable | self | |
integer | last_damage_taken | |
string | last_bone_damaged | |
DamageType | damage_type_reason | |
Vector | hit_from_direction | |
Player or nil | instigator | |
Base Actor or nil | causer | The object which caused the damage |
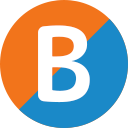
TakeDamage
Triggered when this Entity takes damage
Returnfalse
to cancel the damage (will still display animations, particles and apply impact forces)
Damageable.Subscribe("TakeDamage", function(self, damage, bone, type, from_direction, instigator, causer)
-- TakeDamage was called
end)
Type | Argument | Description |
---|---|---|
Damageable | self | |
integer | damage | |
string | bone | Damaged bone |
DamageType | type | Damage Type |
Vector | from_direction | Direction of the damage relative to the damaged actor |
Player | instigator | The player which caused the damage |
any | causer | The object which caused the damage |