File
A File represents an entry to a system file.
It is not possible to open files from outside the server folder. All path must be relative to the Server's executable folder. All files are opened as binary file by default.
Examples​
local configuration_file = File("my_awesome_configuration.json")
local configuration_file_json = JSON.parse(configuration_file:Read(configuration_file:Size()))
Constructors​
Default Constructor​
local my_file = File(file_path, truncate?)
Type | Name | Default | Description |
---|---|---|---|
string | file_path | If on Server, this is the Path relative to server executable. Otherwise if on Client, this is the Path relative to Client's 'Packages/.transient/' folder | |
boolean | truncate | false | Whether or not to clear the file upon opening it |
Static Functions​
Returns | Name | Description | |
---|---|---|---|
![]() | integer | Time | Returns when a file was last modified in Unix time |
![]() | boolean | CreateDirectory | Creates a Directory (for every folder passed) |
![]() | integer | Remove | Deletes a folder or file |
![]() | boolean | Exists | Verifies if a entry exists in the file system |
![]() | boolean | IsDirectory | Checks if a path is a directory |
![]() | boolean | IsRegularFile | Checks if a path is a file |
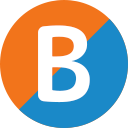
Time
​
Returns when a file was last modified in Unix time
— Returns integer (the last update time in unix time).
local ret = File.Time(path)
Type | Parameter | Default | Description |
---|---|---|---|
string | path | Path to file |
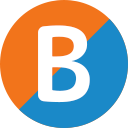
CreateDirectory
​
Creates a Directory (for every folder passed)
— Returns boolean (if succeeded).
local ret = File.CreateDirectory(path)
Type | Parameter | Default | Description |
---|---|---|---|
string | path | Path to folder |
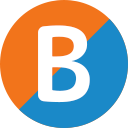
Remove
​
Deletes a folder or file
— Returns integer (amount of files deleted).
local ret = File.Remove(path)
Type | Parameter | Default | Description |
---|---|---|---|
string | path | Path to file or folder |
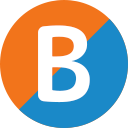
Exists
​
Verifies if a entry exists in the file system
— Returns boolean (if exists).
local ret = File.Exists(path)
Type | Parameter | Default | Description |
---|---|---|---|
string | path | Path to file or folder |
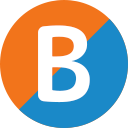
IsDirectory
​
Checks if a path is a directory
— Returns boolean (if is a directory).
local ret = File.IsDirectory(path)
Type | Parameter | Default | Description |
---|---|---|---|
string | path | Path to folder |
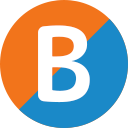
IsRegularFile
​
Checks if a path is a file
— Returns boolean (if is a regular file).
local ret = File.IsRegularFile(path)
Type | Parameter | Default | Description |
---|---|---|---|
string | path | Path to file |
Functions​
Returns | Name | Description | |
---|---|---|---|
![]() | Close | Closes the file and destroys the entity | |
![]() | Flush | Flushes content to the file | |
![]() | boolean | IsEOF | Checks if the file status is End of File |
![]() | boolean | IsBad | Checks if the file status is Bad |
![]() | boolean | IsGood | Checks if the file status is Good |
![]() | boolean | HasFailed | Checks if the last operation has Failed |
![]() | string | Read | Reads characters from the File and returns it. Also moves the file pointer to the latest read position. Pass 0 to read the whole file |
![]() | ReadAsync | Reads characters from the File asynchronously. | |
![]() | string | ReadLine | Reads and returns the next file line |
![]() | table | ReadJSON | Reads the whole file as a JSON and returns it. |
![]() | ReadJSONAsync | Reads the whole file as a JSON and returns it asynchronously. | |
![]() | Seek | Sets the file pointer to a specific position | |
![]() | integer | Size | Returns the size of the file |
![]() | Skip | Skips n (amount) positions from the current file pointer position | |
![]() | integer | Tell | Returns the current file pointer position |
![]() | Write | Writes the Data at the current position of the file |
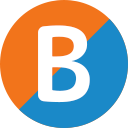
Close
​
Closes the file and destroys the entity
my_file:Close()
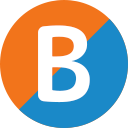
Flush
​
Flushes content to the file
my_file:Flush()
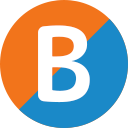
IsEOF
​
Checks if the file status is End of File
— Returns boolean (if is EOF).
local ret = my_file:IsEOF()
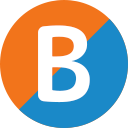
IsBad
​
Checks if the file status is Bad
— Returns boolean (if status is Bad).
local ret = my_file:IsBad()
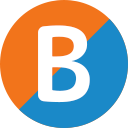
IsGood
​
Checks if the file status is Good
— Returns boolean (if status is Good).
local ret = my_file:IsGood()
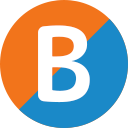
HasFailed
​
Checks if the last operation has Failed
— Returns boolean (if last operation failed).
local ret = my_file:HasFailed()
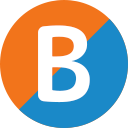
Read
​
Reads characters from the File and returns it. Also moves the file pointer to the latest read position. Pass 0 to read the whole file
— Returns string (file data).
local ret = my_file:Read(length?)
Type | Parameter | Default | Description |
---|---|---|---|
integer | length? | 0 | Length to be read from file |
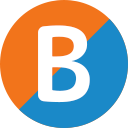
ReadAsync
​
Reads characters from the File asynchronously.
my_file:ReadAsync(length, callback)
Type | Parameter | Default | Description |
---|---|---|---|
integer | length | Length to be read from file | |
function | callback | Callback with this format |
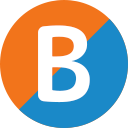
ReadLine
​
Reads and returns the next file line
— Returns string (file line data).
local ret = my_file:ReadLine()
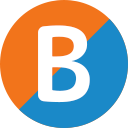
ReadJSON
​
Reads the whole file as a JSON and returns it.
— Returns table (parsed table).
local ret = my_file:ReadJSON()
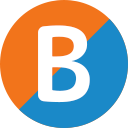
ReadJSONAsync
​
Reads the whole file as a JSON and returns it asynchronously.
my_file:ReadJSONAsync(callback)
Type | Parameter | Default | Description |
---|---|---|---|
function | callback | Callback with the file read with this format |
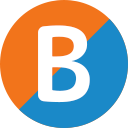
Seek
​
Sets the file pointer to a specific position
my_file:Seek(position)
Type | Parameter | Default | Description |
---|---|---|---|
integer | position | Position to offset the file pointer |
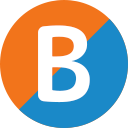
Size
​
Returns the size of the file
— Returns integer (file size).
local ret = my_file:Size()
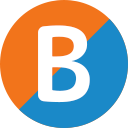
Skip
​
Skips n (amount) positions from the current file pointer position
my_file:Skip(amount)
Type | Parameter | Default | Description |
---|---|---|---|
integer | amount | Amount to offset the file pointer |
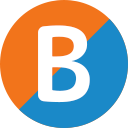
Tell
​
Returns the current file pointer position
— Returns integer (current file pointer position).
local ret = my_file:Tell()
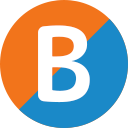
Write
​
Writes the Data at the current position of the file
my_file:Write(data)
Type | Parameter | Default | Description |
---|---|---|---|
string | data | Data to write to the file |