HCharacter
Characters represents Actors which can be possessed, can move and interact with world. They are the default Skeletal Mesh Character built for HELIX.
💂Authority
This class can only be spawned on 🟦 Server side.
👪Inheritance
HCharacters are Skeletal Meshes using Unreal's Metahuman Skeleton, with animations and interactivity already natively integrated into HELIX. It is possible to import any Skeletal Mesh (which uses Unreal's Metahuman Skeleton to this HCharacter.
Examples​
Server/Index.lua
-------------------------------------------------------------------------------------
-- The following example spawns a HCharacter with the Helix Customizable character --
-- when players join the server and destroys them when they leave. --
-------------------------------------------------------------------------------------
-- Function to spawn a Character to a player
function SpawnCharacter(player)
Console.Log("Spawn player")
local new_character = HCharacter(Vector(0, 0, 0), Rotator(0, 0, 0), player)
-- Possess the new Character
player:Possess(new_character)
end
-- Subscribes to an Event which is triggered when Players join the server (i.e. Spawn)
Player.Subscribe("Spawn", SpawnCharacter)
-- Iterates for all already connected players and give them a Character as well
-- This will make sure you also get a Character when you reload the package
Package.Subscribe("Load", function()
for k, player in pairs(Player.GetAll()) do
SpawnCharacter(player)
end
end)
-- When Player leaves the server, destroy it's Character
Player.Subscribe("Destroy", function(player)
local character = player:GetControlledCharacter()
if (character) then
character:Destroy()
end
end)
Constructors​
Default Constructor (Helix Avatar)​
local my_hcharacter = HCharacter(location, rotation, player, collision_type?, gravity_enabled?, max_health?, death_sound?, pain_sound?)
Type | Name | Default | Description |
---|---|---|---|
Vector | location | ||
Rotator | rotation | ||
Player | player | ||
CollisionType | collision_type | CollisionType.Normal | |
boolean | gravity_enabled | true | |
integer | max_health | 100 | Current / Max Health |
Sound Reference | death_sound | helix::A_Male_01_Death | Played when Character dies |
Sound Reference | pain_sound | helix::A_Male_01_Pain | Played when Character takes damage |
Custom Mesh Constructor​
local my_hcharacter = HCharacter(location, rotation, skeletal_mesh, collision_type?, gravity_enabled?, max_health?, death_sound?, pain_sound?)
Type | Name | Default | Description |
---|---|---|---|
Vector | location | ||
Rotator | rotation | ||
SkeletalMesh Reference | skeletal_mesh | ||
CollisionType | collision_type | CollisionType.Normal | |
boolean | gravity_enabled | true | |
integer | max_health | 100 | Current / Max Health |
Sound Reference | death_sound | helix::A_Male_01_Death | Played when Character dies |
Sound Reference | pain_sound | helix::A_Male_01_Pain | Played when Character takes damage |
Static Functions​
Inherited Entity Static Functions
Base Entityscripting-reference/classes/base-classes/Entity
Returns | Name | Description | |
---|---|---|---|
![]() | table of Base Entity | GetAll | Returns a table containing all Entities of the class this is called on |
![]() | Base Entity | GetByIndex | Returns a specific Entity of this class at an index |
![]() | integer | GetCount | Returns how many Entities of this class exist |
![]() | iterator | GetPairs | Returns an iterator with all Entities of this class to be used with pairs() |
![]() | table | Inherit | Inherits this class with the Inheriting System |
![]() | table of table | GetInheritedClasses | Gets a list of all directly inherited classes from this Class created with the Inheriting System |
![]() | table or nil | GetParentClass | Gets the parent class if this Class was created with the Inheriting System |
![]() | boolean | IsChildOf | Gets if this Class is child of another class if this Class was created with the Inheriting System |
![]() | function | Subscribe | Subscribes to an Event for all entities of this Class |
![]() | function | SubscribeRemote | Subscribes to a custom event called from server |
![]() | Unsubscribe | Unsubscribes all callbacks from this Event in this Class within this Package, or only the callback passed |
Functions​
Inherited Entity Functions
Base Entityscripting-reference/classes/base-classes/Entity
Returns | Name | Description | |
---|---|---|---|
![]() | integer | GetID | Gets the universal network ID of this Entity (same on both client and server) |
![]() | table | GetClass | Gets the class of this entity |
![]() | boolean | IsA | Recursively checks if this entity is inherited from a Class |
![]() | function | Subscribe | Subscribes to an Event on this specific entity |
![]() | function | SubscribeRemote | Subscribes to a custom event called from server on this specific entity |
![]() | Unsubscribe | Unsubscribes all callbacks from this Event in this Entity within this Package, or only the callback passed | |
![]() | SetValue | Sets a Value in this Entity | |
![]() | any | GetValue | Gets a Value stored on this Entity at the given key |
Destroy | Destroys this Entity | ||
![]() | boolean | IsValid | Returns true if this Entity is valid (i.e. wasn't destroyed and points to a valid Entity) |
CallRemoteEvent | Calls a custom remote event directly on this entity to a specific Player | ||
CallRemoteEvent | Calls a custom remote event directly on this entity | ||
BroadcastRemoteEvent | Calls a custom remote event directly on this entity to all Players |
Inherited Actor Functions
Base Actorscripting-reference/classes/base-classes/Actor
Returns | Name | Description | |
---|---|---|---|
![]() | AddImpulse | Applies a force in world world to this Actor | |
AttachTo | Attaches this Actor to any other Actor, optionally at a specific bone | ||
Detach | Detaches this Actor from AttachedTo Actor | ||
SetCollision | Sets this Actor's collision type | ||
SetDimension | Sets this Actor's Dimension | ||
![]() | SetForce | Adds a permanent force to this Actor, set to Vector(0, 0, 0) to cancel | |
SetGravityEnabled | Sets whether gravity is enabled on this Actor | ||
![]() | SetVisibility | Sets whether the actor is visible or not | |
SetHighlightEnabled | Sets whether the highlight is enabled on this Actor, and which highlight index to use | ||
SetOutlineEnabled | Sets whether the outline is enabled on this Actor, and which outline index to use | ||
SetLifeSpan | Sets the time (in seconds) before this Actor is destroyed. After this time has passed, the actor will be automatically destroyed. | ||
SetLocation | Sets this Actor's location in the game world | ||
SetRotation | Sets this Actor's rotation in the game world | ||
SetRelativeLocation | Sets this Actor's relative location in local space (only if this actor is attached) | ||
SetRelativeRotation | Sets this Actor's relative rotation in local space (only if this actor is attached) | ||
SetScale | Sets this Actor's scale | ||
SetNetworkAuthority | Sets the Player to have network authority over this Actor | ||
SetNetworkAuthorityAutoDistributed | Sets if this Actor will auto distribute the network authority between players | ||
![]() | TranslateTo | Smoothly moves this actor to a location over a certain time | |
![]() | RotateTo | Smoothly rotates this actor to an angle over a certain time | |
![]() | boolean | IsBeingDestroyed | Returns true if this Actor is being destroyed |
![]() | boolean | IsVisible | Returns true if this Actor is visible |
![]() | boolean | IsGravityEnabled | Returns true if gravity is enabled on this Actor |
![]() | boolean | IsInWater | Returns true if this Actor is in water |
![]() | boolean | IsNetworkDistributed | Returns true if this Actor is currently network distributed |
![]() | table of Base Actor | GetAttachedEntities | Gets all Actors attached to this Actor |
![]() | Base Actor or nil | GetAttachedTo | Gets the Actor this Actor is attached to |
table | GetBounds | Gets this Actor's bounds | |
![]() | CollisionType | GetCollision | Gets this Actor's collision type |
![]() | Vector | GetLocation | Gets this Actor's location in the game world |
![]() | Vector | GetRelativeLocation | Gets this Actor's Relative Location if it's attached |
Player or nil | GetNetworkAuthority | Gets this Actor's Network Authority Player | |
![]() | Rotator | GetRotation | Gets this Actor's angle in the game world |
![]() | Rotator | GetRelativeRotation | Gets this Actor's Relative Rotation if it's attached |
![]() | Vector | GetForce | Gets this Actor's force (set by SetForce() ) |
![]() | integer | GetDimension | Gets this Actor's dimension |
boolean | HasNetworkAuthority | Returns true if the local Player is currently the Network Authority of this Actor | |
boolean | HasAuthority | Gets if this Actor was spawned by the client side | |
![]() | Vector | GetScale | Gets this Actor's scale |
![]() | Vector | GetVelocity | Gets this Actor's current velocity |
AddActorTag | Adds an Unreal Actor Tag to this Actor | ||
RemoveActorTag | Removes an Unreal Actor Tag from this Actor | ||
table of string | GetActorTags | Gets all Unreal Actor Tags on this Actor | |
boolean | WasRecentlyRendered | Gets if this Actor was recently rendered on screen | |
float | GetDistanceFromCamera | Gets the distance of this Actor from the Camera | |
float | GetScreenPercentage | Gets the percentage of this Actor size in the screen |
Inherited Paintable Functions
Base Paintablescripting-reference/classes/base-classes/Paintable
Returns | Name | Description | |
---|---|---|---|
![]() | SetMaterial | Sets the material at the specified index of this Actor | |
SetMaterialFromCanvas | Sets the material at the specified index of this Actor to a Canvas object | ||
SetMaterialFromSceneCapture | Sets the material at the specified index of this Actor to a SceneCapture object | ||
SetMaterialFromWebUI | Sets the material at the specified index of this Actor to a WebUI object | ||
![]() | ResetMaterial | Resets the material from the specified index to the original one | |
![]() | SetMaterialColorParameter | Sets a Color parameter in this Actor's material | |
![]() | SetMaterialScalarParameter | Sets a Scalar parameter in this Actor's material | |
![]() | SetMaterialTextureParameter | Sets a texture parameter in this Actor's material to an image on disk | |
![]() | SetMaterialVectorParameter | Sets a Vector parameter in this Actor's material | |
![]() | SetPhysicalMaterial | Overrides this Actor's Physical Material with a new one |
Inherited Damageable Functions
Base Damageablescripting-reference/classes/base-classes/Damageable
Returns | Name | Description | |
---|---|---|---|
integer | ApplyDamage | Do damage to this entity | |
![]() | integer | GetHealth | Gets the current health |
![]() | integer | GetMaxHealth | Gets the Max Health |
Respawn | Respawns the Entity, fullying it's Health and moving it to it's Initial Location | ||
SetHealth | Sets the Health of this Entity | ||
SetMaxHealth | Sets the MaxHealth of this Entity |
Returns | Name | Description | |
---|---|---|---|
![]() | AddSkeletalMeshAttached | Spawns and Attaches a SkeletalMesh into this Character | |
![]() | AddStaticMeshAttached | Spawns and Attaches a StaticMesh into this Character | |
Drop | Drops any Pickable the Character is holding | ||
Jump | Triggers this Character to jump | ||
PickUp | Gives a Melee/Grenade/Weapon (Pickable) to the Character | ||
PlayAnimation | Plays an Animation Montage on this character | ||
StopAnimation | Stops an Animation Montage on this character | ||
![]() | RemoveSkeletalMeshAttached | Removes, if existing, a SkeletalMesh from this Character given it's custom ID | |
![]() | RemoveStaticMeshAttached | Removes, if existing, a StaticMesh from this Character given it's custom ID | |
![]() | RemoveAllStaticMeshesAttached | Removes all StaticMeshes attached | |
![]() | RemoveAllSkeletalMeshesAttached | Removes all SkeletalMeshes attached | |
SetDeathSound | Changes the Death sound when Character dies | ||
SetMesh | Changes the Character Mesh on the fly | ||
SetInputEnabled | Enables/Disables Character's Input | ||
SetPainSound | Changes the Pain sound when Character takes damage | ||
SetRagdollMode | Sets Character Ragdoll Mode | ||
SetTeam | Sets a Team which will disable damaging same Team Members | ||
![]() | boolean | IsInRagdollMode | Gets if Character is in ragdoll mode |
![]() | boolean | IsInputEnabled | Gets if has input enabled |
table | GetBoneTransform | Gets a Bone Transform in world space given a bone name | |
![]() | SkeletalMesh Reference | GetMesh | Gets the Skeletal Mesh Asset |
![]() | Player or nil | GetPlayer | Gets the possessing Player |
![]() | integer | GetTeam | Gets the Team |
EnterVehicle | Forces a Character to enter into a vehicle |
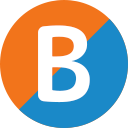
AddSkeletalMeshAttached
​
Spawns and Attaches a SkeletalMesh into this Character, the SkeletalMesh must have the same Skeletal used by the Character Mesh, and will follow all animations from it. Uses a custom ID to be used for removing it further.
For customizing the Materials specific of a SkeletalMeshAttached, please use the following syntax in the Paintable methods:attachable///[ATTACHABLE_ID]/[PARAMETER_NAME]
, where [ATTACHABLE_ID] is the ID of the Attachable, and [PARAMETER_NAME] is the name of the parameter you want to change.
my_hcharacter:AddSkeletalMeshAttached(id, skeletal_mesh_asset?)
Type | Parameter | Default | Description |
---|---|---|---|
string | id | Used further for removing or applying material settings on it | |
SkeletalMesh Reference | skeletal_mesh_asset? |
|
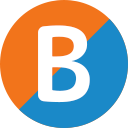
AddStaticMeshAttached
​
Spawns and Attaches a StaticMesh into this Character in a Socket with relative Location and Rotation. Uses a custom ID to be used for removing it further
For customizing the Materials specific of a StaticMeshAttached, please use the following syntax as theparameter_name
in the Paintable methods:attachable///[ATTACHABLE_ID]/[PARAMETER_NAME]
, where [ATTACHABLE_ID] is the ID of the Attachable, and [PARAMETER_NAME] is the name of the parameter you want to change.
my_hcharacter:AddStaticMeshAttached(id, static_mesh_asset?, socket?, relative_location?, relative_rotation?)
Type | Parameter | Default | Description |
---|---|---|---|
string | id | Used further for removing or applying material settings on it | |
StaticMesh Reference | static_mesh_asset? |
| |
string | socket? |
| |
Vector | relative_location? | Vector(0, 0, 0) | |
Rotator | relative_rotation? | Rotator(0, 0, 0) |

Drop
​
Drops any Pickable the Character is holding
my_hcharacter:Drop()

Jump
​
Triggers this Character to jump
my_hcharacter:Jump()

PickUp
​
Gives a Melee/Grenade/Weapon (Pickable) to the Character
my_hcharacter:PickUp(pickable)
Type | Parameter | Default | Description |
---|---|---|---|
Base Pickable | pickable |

PlayAnimation
​
Plays an Animation Montage on this character
my_hcharacter:PlayAnimation(animation_path, slot_type?, loop_indefinitely?, blend_in_time?, blend_out_time?, play_rate?, stop_all_montages?)
Type | Parameter | Default | Description |
---|---|---|---|
Animation Reference | animation_path | ||
AnimationSlotType | slot_type? | AnimationSlotType.FullBody | |
boolean | loop_indefinitely? | false | |
float | blend_in_time? | 0.25 | |
float | blend_out_time? | 0.25 | |
float | play_rate? | 1.0 | |
boolean | stop_all_montages? | false | Stops all running Montages from the same Group |

StopAnimation
​
Stops an Animation Montage on this character
my_hcharacter:StopAnimation(animation_asset)
Type | Parameter | Default | Description |
---|---|---|---|
Animation Reference | animation_asset |
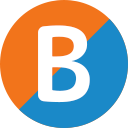
RemoveSkeletalMeshAttached
​
Removes, if existing, a SkeletalMesh from this Character given it's custom ID
my_hcharacter:RemoveSkeletalMeshAttached(id)
Type | Parameter | Default | Description |
---|---|---|---|
string | id |
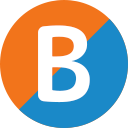
RemoveStaticMeshAttached
​
Removes, if existing, a StaticMesh from this Character given it's custom ID
my_hcharacter:RemoveStaticMeshAttached(id)
Type | Parameter | Default | Description |
---|---|---|---|
string | id |
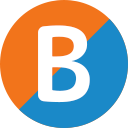
RemoveAllStaticMeshesAttached
​
Removes all StaticMeshes attached
my_hcharacter:RemoveAllStaticMeshesAttached()
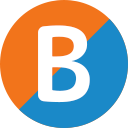
RemoveAllSkeletalMeshesAttached
​
Removes all SkeletalMeshes attached
my_hcharacter:RemoveAllSkeletalMeshesAttached()

SetDeathSound
​
Changes the Death sound when Character dies
my_hcharacter:SetDeathSound(sound_asset)
Type | Parameter | Default | Description |
---|---|---|---|
string | sound_asset |

SetMesh
​
Changes the Character Mesh on the fly
my_hcharacter:SetMesh(skeletal_mesh_asset)
Type | Parameter | Default | Description |
---|---|---|---|
SkeletalMesh Reference | skeletal_mesh_asset |

SetInputEnabled
​
Enables/Disables Character's Input
my_hcharacter:SetInputEnabled(is_enabled)
Type | Parameter | Default | Description |
---|---|---|---|
boolean | is_enabled |

SetPainSound
​
Changes the Pain sound when Character takes damage
my_hcharacter:SetPainSound(sound_asset)
Type | Parameter | Default | Description |
---|---|---|---|
Sound Reference | sound_asset |

SetRagdollMode
​
Sets Character Ragdoll Mode
my_hcharacter:SetRagdollMode(ragdoll_enabled)
Type | Parameter | Default | Description |
---|---|---|---|
boolean | ragdoll_enabled |

SetTeam
​
Sets a Team which will disable damaging same Team Members
my_hcharacter:SetTeam(team)
Type | Parameter | Default | Description |
---|---|---|---|
integer | team | 0 is neutral and default |
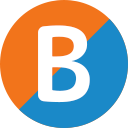
IsInRagdollMode
​
Gets if Character is in ragdoll mode
— Returns boolean.
local ret = my_hcharacter:IsInRagdollMode()
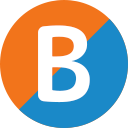
IsInputEnabled
​
Gets if has input enabled
— Returns boolean.
local ret = my_hcharacter:IsInputEnabled()

GetBoneTransform
​
Gets a Bone Transform in world space given a bone name
— Returns table (with this format).
local ret = my_hcharacter:GetBoneTransform(bone_name)
Type | Parameter | Default | Description |
---|---|---|---|
string | bone_name |
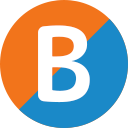
GetMesh
​
Gets the Skeletal Mesh Asset
— Returns SkeletalMesh Reference.
local ret = my_hcharacter:GetMesh()
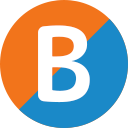
GetPlayer
​
Gets the possessing Player
local ret = my_hcharacter:GetPlayer()
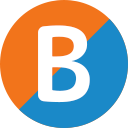
GetTeam
​
Gets the Team
— Returns integer.
local ret = my_hcharacter:GetTeam()

EnterVehicle
​
Forces a Character to enter into a vehicle
my_hcharacter:EnterVehicle(my_HSimpleVehicle, Seat?)
Type | Parameter | Default | Description |
---|---|---|---|
HSimpleVehicle | my_HSimpleVehicle | ||
integer | Seat? | 0 |
Events​
Inherited Entity Events
Base Entityscripting-reference/classes/base-classes/Entity
Name | Description | |
---|---|---|
![]() | Spawn | Triggered when an Entity is spawned/created |
![]() | Destroy | Triggered when an Entity is destroyed |
![]() | ValueChange | Triggered when an Entity has a value changed with :SetValue() |
![]() | ClassRegister | Triggered when a new Class is registered with the Inheriting System |
Inherited Actor Events
Base Actorscripting-reference/classes/base-classes/Actor
Name | Description | |
---|---|---|
![]() | Spawn | Triggered when an Entity is spawned/created |
![]() | Destroy | Triggered when an Entity is destroyed |
![]() | ValueChange | Triggered when an Entity has a value changed with :SetValue() |
![]() | ClassRegister | Triggered when a new Class is registered with the Inheriting System |
Inherited Damageable Events
Base Damageablescripting-reference/classes/base-classes/Damageable
Name | Description | |
---|---|---|
![]() | Spawn | Triggered when an Entity is spawned/created |
![]() | Destroy | Triggered when an Entity is destroyed |
![]() | ValueChange | Triggered when an Entity has a value changed with :SetValue() |
![]() | ClassRegister | Triggered when a new Class is registered with the Inheriting System |
Name | Description | |
---|---|---|
AnimationBeginNotify | When an Animation Montage Notify begins | |
AnimationEndNotify | When an Animation Montage Notify ends | |
![]() | Drop | When Character drops the currently picked up Pickable |
![]() | Fire | When Character fires a Weapon |
![]() | GrabProp | When Character grabs up a Prop |
Interact | Triggered when a Character interacts with a Prop or Pickable | |
![]() | PickUp | When Character picks up anything |
![]() | Possess | When Character is possessed |
![]() | RagdollModeChange | When Character enters or leaves ragdoll |
![]() | AttemptReload | Triggered when this Character attempts to reload |
![]() | Reload | When Character reloads a weapon |
![]() | UnPossess | When Character is unpossessed |
![]() | PullUse | Triggered when a Character presses the use button for a Pickable (i.e. clicks left mouse button with this equipped) |
![]() | ReleaseUse | Triggered when a Character releases the use button for a Pickable (i.e. releases left mouse button with this equipped) |

AnimationBeginNotify
​
When an Animation Montage Notify begins
HCharacter.Subscribe("AnimationBeginNotify", function(self, notify_name)
-- AnimationBeginNotify was called
end)

AnimationEndNotify
​
When an Animation Montage Notify ends
HCharacter.Subscribe("AnimationEndNotify", function(self, notify_name)
-- AnimationEndNotify was called
end)
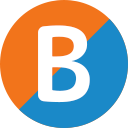
Drop
​
When Character drops the currently picked up Pickable
HCharacter.Subscribe("Drop", function(self, object, triggered_by_player)
-- Drop was called
end)
Type | Argument | Description |
---|---|---|
Character | self | |
Base Pickable | object | |
boolean | triggered_by_player |
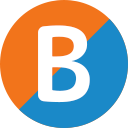
Fire
​
When Character fires a Weapon
HCharacter.Subscribe("Fire", function(self, weapon)
-- Fire was called
end)
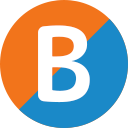
GrabProp
​
When Character grabs up a Prop
HCharacter.Subscribe("GrabProp", function(self, prop)
-- GrabProp was called
end)

Interact
​
Triggered when a Character interacts with a Prop or Pickable
Returnfalse
to prevent it
HCharacter.Subscribe("Interact", function(self, object)
-- Interact was called
end)
Type | Argument | Description |
---|---|---|
Character | self | |
Prop or Base Pickable | object |
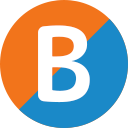
PickUp
​
When Character picks up anything
HCharacter.Subscribe("PickUp", function(self, object)
-- PickUp was called
end)
Type | Argument | Description |
---|---|---|
Character | self | |
Base Pickable | object |
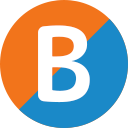
Possess
​
When Character is possessed
HCharacter.Subscribe("Possess", function(self, possesser)
-- Possess was called
end)
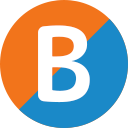
RagdollModeChange
​
When Character enters or leaves ragdoll
HCharacter.Subscribe("RagdollModeChange", function(self, old_state, new_state)
-- RagdollModeChange was called
end)
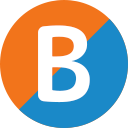
AttemptReload
​
Triggered when this Character attempts to reload
Returnfalse
to prevent it
HCharacter.Subscribe("AttemptReload", function(self, weapon)
-- AttemptReload was called
end)
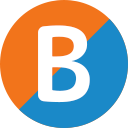
Reload
​
When Character reloads a weapon
HCharacter.Subscribe("Reload", function(self, weapon, ammo_to_reload)
-- Reload was called
end)
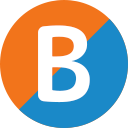
UnPossess
​
When Character is unpossessed
HCharacter.Subscribe("UnPossess", function(self, old_possesser)
-- UnPossess was called
end)
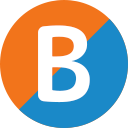
PullUse
​
Triggered when a Character presses the use button for a Pickable (i.e. clicks left mouse button with this equipped)
HCharacter.Subscribe("PullUse", function(self, pickable)
-- PullUse was called
end)
Type | Argument | Description |
---|---|---|
Character | self | The Character that used it |
Base Pickable | pickable | The Pickable which has just been used |
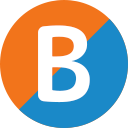
ReleaseUse
​
Triggered when a Character releases the use button for a Pickable (i.e. releases left mouse button with this equipped)
HCharacter.Subscribe("ReleaseUse", function(self, pickable)
-- ReleaseUse was called
end)
Type | Argument | Description |
---|---|---|
Character | self | The Character that stopped using it |
Base Pickable | pickable | The Pickable which has just stopped being used |