Character
Characters represents Actors which can be possessed, can move and interact with world. They are the default Skeletal Mesh Character built for HELIX.
Characters are Skeletal Meshes using Unreal's Mannequin Skeletal, with animations and interactivity already natively integrated into HELIX. It is possible to import any Skeletal Mesh (which uses Unreal's Mannequin Skeletal) to this Character.
Examples​
-- The following examples are using all Skeletal Meshes which we currently have:
local male = Character(Vector(200, 0, 100), Rotator(0, 0, 0), "helix::SK_Male")
local manny = Character(Vector(300, 0, 100), Rotator(0, 0, 0), "helix::SK_Manny")
local quinn = Character(Vector(400, 0, 100), Rotator(0, 0, 0), "helix::SK_Quinn")
-- Adds head to Male
new_char:AddSkeletalMeshAttached("head", "helix::SK_Male_Head")
More related examples:
Play as Propgetting-started/code-examples/play-as-propNametagsgetting-started/code-examples/name-tagsConstructors​
Default Constructor​
local my_character = Character(location, rotation, skeletal_mesh, collision_type?, gravity_enabled?, max_health?, death_sound?, pain_sound?)
Type | Name | Default | Description |
---|---|---|---|
Vector | location | ||
Rotator | rotation | ||
SkeletalMesh Reference | skeletal_mesh | ||
CollisionType | collision_type | CollisionType.Normal | |
boolean | gravity_enabled | true | |
integer | max_health | 100 | Current / Max Health |
Sound Reference | death_sound | helix::A_Male_01_Death | Played when Character dies |
Sound Reference | pain_sound | helix::A_Male_01_Pain | Played when Character takes damage |
Static Functions​
Inherited Entity Static Functions
Returns | Name | Description | |
---|---|---|---|
![]() | table of Base Entity | GetAll | Returns a table containing all Entities of the class this is called on |
![]() | Base Entity | GetByIndex | Returns a specific Entity of this class at an index |
![]() | integer | GetCount | Returns how many Entities of this class exist |
![]() | iterator | GetPairs | Returns an iterator with all Entities of this class to be used with pairs() |
![]() | table | Inherit | Inherits this class with the Inheriting System |
![]() | table of table | GetInheritedClasses | Gets a list of all directly inherited classes from this Class created with the Inheriting System |
![]() | table or nil | GetParentClass | Gets the parent class if this Class was created with the Inheriting System |
![]() | boolean | IsChildOf | Gets if this Class is child of another class if this Class was created with the Inheriting System |
![]() | function | Subscribe | Subscribes to an Event for all entities of this Class |
![]() | function | SubscribeRemote | Subscribes to a custom event called from server |
![]() | Unsubscribe | Unsubscribes all callbacks from this Event in this Class within this Package, or only the callback passed |
Functions​
Inherited Entity Functions
Returns | Name | Description | |
---|---|---|---|
![]() | integer | GetID | Gets the universal network ID of this Entity (same on both client and server) |
![]() | table | GetClass | Gets the class of this entity |
![]() | boolean | IsA | Recursively checks if this entity is inherited from a Class |
![]() | function | Subscribe | Subscribes to an Event on this specific entity |
![]() | function | SubscribeRemote | Subscribes to a custom event called from server on this specific entity |
![]() | Unsubscribe | Unsubscribes all callbacks from this Event in this Entity within this Package, or only the callback passed | |
![]() | SetValue | Sets a Value in this Entity | |
![]() | any | GetValue | Gets a Value stored on this Entity at the given key |
Destroy | Destroys this Entity | ||
![]() | boolean | IsValid | Returns true if this Entity is valid (i.e. wasn't destroyed and points to a valid Entity) |
CallRemoteEvent | Calls a custom remote event directly on this entity to a specific Player | ||
CallRemoteEvent | Calls a custom remote event directly on this entity | ||
BroadcastRemoteEvent | Calls a custom remote event directly on this entity to all Players |
Inherited Actor Functions
Returns | Name | Description | |
---|---|---|---|
![]() | AddImpulse | Applies a force in world world to this Actor | |
AttachTo | Attaches this Actor to any other Actor, optionally at a specific bone | ||
Detach | Detaches this Actor from AttachedTo Actor | ||
SetCollision | Sets this Actor's collision type | ||
SetDimension | Sets this Actor's Dimension | ||
![]() | SetForce | Adds a permanent force to this Actor, set to Vector(0, 0, 0) to cancel | |
SetGravityEnabled | Sets whether gravity is enabled on this Actor | ||
![]() | SetVisibility | Sets whether the actor is visible or not | |
SetHighlightEnabled | Sets whether the highlight is enabled on this Actor, and which highlight index to use | ||
SetOutlineEnabled | Sets whether the outline is enabled on this Actor, and which outline index to use | ||
SetLifeSpan | Sets the time (in seconds) before this Actor is destroyed. After this time has passed, the actor will be automatically destroyed. | ||
SetLocation | Sets this Actor's location in the game world | ||
SetRotation | Sets this Actor's rotation in the game world | ||
SetRelativeLocation | Sets this Actor's relative location in local space (only if this actor is attached) | ||
SetRelativeRotation | Sets this Actor's relative rotation in local space (only if this actor is attached) | ||
SetScale | Sets this Actor's scale | ||
SetNetworkAuthority | Sets the Player to have network authority over this Actor | ||
SetNetworkAuthorityAutoDistributed | Sets if this Actor will auto distribute the network authority between players | ||
![]() | TranslateTo | Smoothly moves this actor to a location over a certain time | |
![]() | RotateTo | Smoothly rotates this actor to an angle over a certain time | |
![]() | boolean | IsBeingDestroyed | Returns true if this Actor is being destroyed |
![]() | boolean | IsVisible | Returns true if this Actor is visible |
![]() | boolean | IsGravityEnabled | Returns true if gravity is enabled on this Actor |
![]() | boolean | IsInWater | Returns true if this Actor is in water |
![]() | boolean | IsNetworkDistributed | Returns true if this Actor is currently network distributed |
![]() | table of Base Actor | GetAttachedEntities | Gets all Actors attached to this Actor |
![]() | Base Actor or nil | GetAttachedTo | Gets the Actor this Actor is attached to |
table | GetBounds | Gets this Actor's bounds | |
![]() | CollisionType | GetCollision | Gets this Actor's collision type |
![]() | Vector | GetLocation | Gets this Actor's location in the game world |
![]() | Vector | GetRelativeLocation | Gets this Actor's Relative Location if it's attached |
Player or nil | GetNetworkAuthority | Gets this Actor's Network Authority Player | |
![]() | Rotator | GetRotation | Gets this Actor's angle in the game world |
![]() | Rotator | GetRelativeRotation | Gets this Actor's Relative Rotation if it's attached |
![]() | Vector | GetForce | Gets this Actor's force (set by SetForce() ) |
![]() | integer | GetDimension | Gets this Actor's dimension |
boolean | HasNetworkAuthority | Returns true if the local Player is currently the Network Authority of this Actor | |
boolean | HasAuthority | Gets if this Actor was spawned by the client side | |
![]() | Vector | GetScale | Gets this Actor's scale |
![]() | Vector | GetVelocity | Gets this Actor's current velocity |
AddActorTag | Adds an Unreal Actor Tag to this Actor | ||
RemoveActorTag | Removes an Unreal Actor Tag from this Actor | ||
table of string | GetActorTags | Gets all Unreal Actor Tags on this Actor | |
boolean | WasRecentlyRendered | Gets if this Actor was recently rendered on screen | |
float | GetDistanceFromCamera | Gets the distance of this Actor from the Camera | |
float | GetScreenPercentage | Gets the percentage of this Actor size in the screen |
Inherited Paintable Functions
Returns | Name | Description | |
---|---|---|---|
![]() | SetMaterial | Sets the material at the specified index of this Actor | |
SetMaterialFromCanvas | Sets the material at the specified index of this Actor to a Canvas object | ||
SetMaterialFromSceneCapture | Sets the material at the specified index of this Actor to a SceneCapture object | ||
SetMaterialFromWebUI | Sets the material at the specified index of this Actor to a WebUI object | ||
![]() | ResetMaterial | Resets the material from the specified index to the original one | |
![]() | SetMaterialColorParameter | Sets a Color parameter in this Actor's material | |
![]() | SetMaterialScalarParameter | Sets a Scalar parameter in this Actor's material | |
![]() | SetMaterialTextureParameter | Sets a texture parameter in this Actor's material to an image on disk | |
![]() | SetMaterialVectorParameter | Sets a Vector parameter in this Actor's material | |
![]() | SetPhysicalMaterial | Overrides this Actor's Physical Material with a new one |
Inherited Damageable Functions
Returns | Name | Description | |
---|---|---|---|
integer | ApplyDamage | Do damage to this entity | |
![]() | integer | GetHealth | Gets the current health |
![]() | integer | GetMaxHealth | Gets the Max Health |
Respawn | Respawns the Entity, fullying it's Health and moving it to it's Initial Location | ||
SetHealth | Sets the Health of this Entity | ||
SetMaxHealth | Sets the MaxHealth of this Entity |
Returns | Name | Description | |
---|---|---|---|
![]() | AddSkeletalMeshAttached | Spawns and Attaches a SkeletalMesh into this Character | |
![]() | AddStaticMeshAttached | Spawns and Attaches a StaticMesh into this Character | |
Drop | Drops any Pickable the Character is holding | ||
EnterVehicle | Enters the Vehicle at Seat (0 - Driver) | ||
GrabProp | Gives a Prop to the Character | ||
![]() | HideBone | Hides a bone of this Character | |
![]() | UnHideBone | UnHide a bone of this Character | |
![]() | boolean | IsBoneHidden | Gets if a bone is hidden |
Jump | Triggers this Character to jump | ||
LeaveVehicle | Leaves the current Vehicle | ||
LookAt | AI: Tries to make this Character to look at Location | ||
MoveTo | AI: Makes this Character to walk to the Location | ||
Follow | AI: Makes this Character to follow another actor | ||
StopMovement | AI: Stops the movement | ||
PickUp | Gives a Melee/Grenade/Weapon (Pickable) to the Character | ||
PlayAnimation | Plays an Animation Montage on this character | ||
StopAnimation | Stops an Animation Montage on this character | ||
![]() | RemoveSkeletalMeshAttached | Removes, if existing, a SkeletalMesh from this Character given it's custom ID | |
![]() | RemoveStaticMeshAttached | Removes, if existing, a StaticMesh from this Character given it's custom ID | |
![]() | RemoveAllStaticMeshesAttached | Removes all StaticMeshes attached | |
![]() | RemoveAllSkeletalMeshesAttached | Removes all SkeletalMeshes attached | |
SetAnimationIdleWalkRunStanding | Sets the Blend Space (2D) Animation for Standing | ||
SetAnimationIdleWalkRunCrouching | Sets the Blend Space (2D) Animation for Crouching Horizontal Axis stands for Speed X and Vertical Axis for Speed Y | ||
SetAnimationIdleWalkRunProning | Sets the Blend Space (2D) Animation for Proning Horizontal Axis stands for Speed X and Vertical Axis for Speed Y | ||
SetAnimationsTransitionStandingCrouching | Sets the Transition Animation between Standing and Crouching | ||
SetAnimationsTransitionCrouchingProning | Sets the Transition Animation between Crouching and Proning | ||
SetAccelerationSettings | Sets the Movement Max Acceleration of this Character | ||
SetBrakingSettings | Sets the Movement Braking Settings of this Character | ||
SetCameraMode | Sets the Camera Mode | ||
SetCameraOffset | Sets the Camera Offset (only affects TPS) | ||
SetCanCrouch | Sets if this Character is allowed to Crouch and to Prone | ||
SetCanAim | Sets if this Character is allowed to Aim | ||
SetCanDrop | Sets if this Character is allowed to Drop the Picked up item | ||
SetCanJump | Sets if this Character is allowed to Jump | ||
SetCanDive | Sets if this Character is allowed to Dive | ||
SetCanSprint | Sets if this Character is allowed to Sprint | ||
SetCanGrabProps | Sets if this Character is allowed to Grab any Prop | ||
SetCanPickupPickables | Sets if this Character is allowed to Pick up any Pickable (Weapon, Grenade, Melee...) | ||
SetCanPunch | Sets if this Character is allowed to Punch | ||
SetCanDeployParachute | Sets if this Character is allowed to deploy the Parachute | ||
SetCapsuleSize | Sets this Character's Capsule size | ||
SetDamageMultiplier | Changes how much damage this character takes on specific bones | ||
SetDeathSound | Changes the Death sound when Character dies | ||
SetFallDamageTaken | Set the Fall Damage multiplier taken when falling from High places | ||
SetFlyingMode | Sets the Flying Mode | ||
SetFOVMultiplier | Sets the Field of View multiplier | ||
SetHighFallingTime | Sets time to transition to HighFalling state | ||
SetGaitMode | Sets the Gait Mode | ||
SetGravityScale | Changes the Gravity Scale | ||
SetImpactDamageTaken | Set the Impact Damage taken when being roamed by things | ||
SetRadialDamageToRagdoll | Set the minimum radial damage (e.g. explosions) taken to enter in ragdoll mode | ||
SetFootstepVolumeMultiplier | Set the Footstep Volume multiplier | ||
SetInvulnerable | Sets if the Character can receive any damage | ||
SetJumpZVelocity | Sets the velocity of the jump | ||
SetMesh | Changes the Character Mesh on the fly | ||
![]() | SetMorphTarget | Set Morph Target with Name and Value | |
![]() | float | GetMorphTarget | Returns the value of a Morph Target |
![]() | ClearMorphTargets | Clear all Morph Target that are set to this Mesh | |
table of string | GetAllMorphTargetNames | Returns a table with all morph targets available | |
![]() | SetPhysicalAnimationSettings | Applies the physical animation settings to the body given | |
![]() | ResetPhysicalAnimationSettings | Resets all Physical Animation settings | |
SetInputEnabled | Enables/Disables Character's Input | ||
SetParachuteTexture | Changes the Parachute Texture | ||
SetPainSound | Changes the Pain sound when Character takes damage | ||
SetPunchDamage | Set the Punch Damage this Character will apply on others | ||
SetRagdollMode | Sets Character Ragdoll Mode | ||
SetSimulatePhysics | Sets the Character Capsule to simulate physics | ||
SetSpeedMultiplier | Sets all speed multiplier | ||
SetStanceMode | Sets the Stance Mode | ||
SetTeam | Sets a Team which will disable damaging same Team Members | ||
![]() | SetViewMode | Sets the View Mode | |
![]() | SetWeaponAimMode | Sets the Weapon's Aim Mode | |
UnGrabProp | UnGrabs/Drops the Prop the Character is holding | ||
![]() | boolean | IsInRagdollMode | Gets if Character is in ragdoll mode |
![]() | boolean | IsInvulnerable | Gets if is invulnerable |
![]() | boolean | IsInputEnabled | Gets if has input enabled |
![]() | CameraMode | GetCameraMode | Gets the camera mode |
![]() | boolean | GetCanDrop | Gets if can drop |
![]() | boolean | GetCanPunch | Gets if can punch |
![]() | boolean | GetCanAim | Gets if can aim |
![]() | boolean | GetCanCrouch | Gets if can crouch |
![]() | boolean | GetCanSprint | Gets if can sprint |
![]() | boolean | GetCanGrabProps | Gets if can grab props |
![]() | boolean | GetCanPickupPickables | Gets if can pickup Pickables (Weapons, Melee, Grenade...) |
![]() | table | GetCapsuleSize | Gets the Capsule Size |
table | GetBoneTransform | Gets a Bone Transform in world space given a bone name | |
![]() | Rotator | GetControlRotation | Gets the Control Rotation |
![]() | float | GetDamageMultiplier | Gets the Damage Multiplier of a bone |
![]() | integer | GetFallDamageTaken | Gets the Fall Damage |
![]() | FallingMode | GetFallingMode | Gets the FallingMode |
![]() | boolean | GetFlyingMode | Gets if it's in Flying mode |
![]() | GaitMode | GetGaitMode | Gets the GaitMode |
![]() | Prop or nil | GetGrabbedProp | Gets the Grabbed Prop |
![]() | float | GetGravityScale | Gets the gravity scale |
![]() | integer | GetImpactDamageTaken | Gets the impact damage taken |
![]() | integer | GetJumpZVelocity | Gets the Jump Z Velocity |
![]() | SkeletalMesh Reference | GetMesh | Gets the Skeletal Mesh Asset |
![]() | Vector | GetMovingTo | Gets the Moving To location |
![]() | Base Pickable or nil | GetPicked | Gets the Pickable if picking up |
![]() | Player or nil | GetPlayer | Gets the possessing Player |
![]() | integer | GetPunchDamage | Gets the punch damage |
![]() | float | GetSpeedMultiplier | Gets the speed multiplier |
![]() | StanceMode | GetStanceMode | Gets the Stance Mode |
![]() | SwimmingMode | GetSwimmingMode | Gets the Swimming Mode |
![]() | integer | GetTeam | Gets the Team |
![]() | Vehicle or nil | GetVehicle | Gets the entered Vehicle |
![]() | ViewMode | GetViewMode | Gets the View Mode |
![]() | AimMode | GetWeaponAimMode | Gets the Weapon Aim Mode |
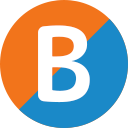
AddSkeletalMeshAttached
​
Spawns and Attaches a SkeletalMesh into this Character, the SkeletalMesh must have the same Skeletal used by the Character Mesh, and will follow all animations from it. Uses a custom ID to be used for removing it further.
For customizing the Materials specific of a SkeletalMeshAttached, please use the following syntax in the Paintable methods:attachable///[ATTACHABLE_ID]/[PARAMETER_NAME]
, where [ATTACHABLE_ID] is the ID of the Attachable, and [PARAMETER_NAME] is the name of the parameter you want to change.
my_character:AddSkeletalMeshAttached(id, skeletal_mesh_asset?)
Type | Parameter | Default | Description |
---|---|---|---|
string | id | Used further for removing or applying material settings on it | |
SkeletalMesh Reference | skeletal_mesh_asset? |
|
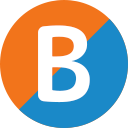
AddStaticMeshAttached
​
Spawns and Attaches a StaticMesh into this Character in a Socket with relative Location and Rotation. Uses a custom ID to be used for removing it further
For customizing the Materials specific of a StaticMeshAttached, please use the following syntax as theparameter_name
in the Paintable methods:attachable///[ATTACHABLE_ID]/[PARAMETER_NAME]
, where [ATTACHABLE_ID] is the ID of the Attachable, and [PARAMETER_NAME] is the name of the parameter you want to change.
my_character:AddStaticMeshAttached(id, static_mesh_asset?, socket?, relative_location?, relative_rotation?)
Type | Parameter | Default | Description |
---|---|---|---|
string | id | Used further for removing or applying material settings on it | |
StaticMesh Reference | static_mesh_asset? |
| |
string | socket? |
| |
Vector | relative_location? | Vector(0, 0, 0) | |
Rotator | relative_rotation? | Rotator(0, 0, 0) |

Drop
​
Drops any Pickable the Character is holding
my_character:Drop()

EnterVehicle
​
Enters the Vehicle at Seat (0 - Driver)
my_character:EnterVehicle(vehicle, seat?)

GrabProp
​
Gives a Prop to the Character
my_character:GrabProp(prop)
Type | Parameter | Default | Description |
---|---|---|---|
Prop | prop |
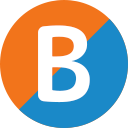
HideBone
​
Hides a bone of this Character.
Check Bone Names List
my_character:HideBone(bone_name?)
Type | Parameter | Default | Description |
---|---|---|---|
string | bone_name? |
| Bone to hide |
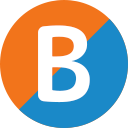
UnHideBone
​
UnHide a bone of this Character.
Check Bone Names List
my_character:UnHideBone(bone_name)
Type | Parameter | Default | Description |
---|---|---|---|
string | bone_name | Bone to unhide |
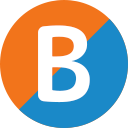
IsBoneHidden
​
Gets if a bone is hidden
— Returns boolean (if the bone is hidden).
local ret = my_character:IsBoneHidden(bone_name)
Type | Parameter | Default | Description |
---|---|---|---|
string | bone_name | Bone to check |

Jump
​
Triggers this Character to jump
my_character:Jump()

LeaveVehicle
​
Leaves the current Vehicle
my_character:LeaveVehicle()

LookAt
​
AI: Tries to make this Character to look at Location
my_character:LookAt(location)
Type | Parameter | Default | Description |
---|---|---|---|
Vector | location | World location to look |

MoveTo
​
AI: Makes this Character to walk to the Location
Triggers event MoveComplete
my_character:MoveTo(location, acceptance_radius?)

Follow
​
AI: Makes this Character to follow another actor
Triggers event MoveComplete
my_character:Follow(actor, acceptance_radius?, stop_on_succeed?, stop_on_fail?, update_rate?)
Type | Parameter | Default | Description |
---|---|---|---|
Base Actor | actor | Actor to follow | |
float | acceptance_radius? | 50 | Radius to consider success |
boolean | stop_on_succeed? | false | Whether to stop when reaching the target |
boolean | stop_on_fail? | false | Whether to stop when failed to reach the target |
float | update_rate? | 0.25 | How often to recalculate the AI path |

StopMovement
​
AI: Stops the movement
Triggers event [MoveCompleted](character#movecompleted)
my_character:StopMovement()

PickUp
​
Gives a Melee/Grenade/Weapon (Pickable) to the Character
my_character:PickUp(pickable)
Type | Parameter | Default | Description |
---|---|---|---|
Base Pickable | pickable |

PlayAnimation
​
Plays an Animation Montage on this character
my_character:PlayAnimation(animation_path, slot_type?, loop_indefinitely?, blend_in_time?, blend_out_time?, play_rate?, stop_all_montages?)
Type | Parameter | Default | Description |
---|---|---|---|
Animation Reference | animation_path | ||
AnimationSlotType | slot_type? | AnimationSlotType.FullBody | |
boolean | loop_indefinitely? | false | |
float | blend_in_time? | 0.25 | |
float | blend_out_time? | 0.25 | |
float | play_rate? | 1.0 | |
boolean | stop_all_montages? | false | Stops all running Montages from the same Group |

StopAnimation
​
Stops an Animation Montage on this character
my_character:StopAnimation(animation_asset)
Type | Parameter | Default | Description |
---|---|---|---|
Animation Reference | animation_asset |
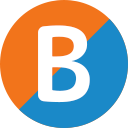
RemoveSkeletalMeshAttached
​
Removes, if existing, a SkeletalMesh from this Character given it's custom ID
my_character:RemoveSkeletalMeshAttached(id)
Type | Parameter | Default | Description |
---|---|---|---|
string | id |
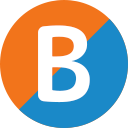
RemoveStaticMeshAttached
​
Removes, if existing, a StaticMesh from this Character given it's custom ID
my_character:RemoveStaticMeshAttached(id)
Type | Parameter | Default | Description |
---|---|---|---|
string | id |
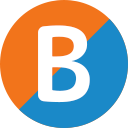
RemoveAllStaticMeshesAttached
​
Removes all StaticMeshes attached
my_character:RemoveAllStaticMeshesAttached()
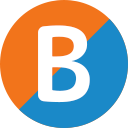
RemoveAllSkeletalMeshesAttached
​
Removes all SkeletalMeshes attached
my_character:RemoveAllSkeletalMeshesAttached()

SetAnimationIdleWalkRunStanding
​
Sets the Blend Space (2D) Animation for Standing
Horizontal Axis stands for Speed X and Vertical Axis for Speed Y
my_character:SetAnimationIdleWalkRunStanding(blend_space_path, enable_turn_in_place?)

SetAnimationIdleWalkRunCrouching
​
Sets the Blend Space (2D) Animation for Crouching
Horizontal Axis stands for Speed X and Vertical Axis for Speed Y
my_character:SetAnimationIdleWalkRunCrouching(blend_space_path, enable_turn_in_place?)

SetAnimationIdleWalkRunProning
​
Sets the Blend Space (2D) Animation for Proning
Horizontal Axis stands for Speed X and Vertical Axis for Speed Y
my_character:SetAnimationIdleWalkRunProning(blend_space_path)
Type | Parameter | Default | Description |
---|---|---|---|
Animation | blend_space_path |

SetAnimationsTransitionStandingCrouching
​
Sets the Transition Animation between Standing and Crouching
my_character:SetAnimationsTransitionStandingCrouching(standing_to_crouching, crouching_to_standing)

SetAnimationsTransitionCrouchingProning
​
Sets the Transition Animation between Crouching and Proning
my_character:SetAnimationsTransitionCrouchingProning(crouching_to_proning, pronng_to_crouching)

SetAccelerationSettings
​
Sets the Movement Max Acceleration of this Character
my_character:SetAccelerationSettings(walking?, parachuting?, skydiving?, falling?, swimming?, swimming_surface?, flying?)
Type | Parameter | Default | Description |
---|---|---|---|
integer | walking? | 768 | |
integer | parachuting? | 512 | |
integer | skydiving? | 768 | |
integer | falling? | 128 | |
integer | swimming? | 256 | |
integer | swimming_surface? | 256 | |
integer | flying? | 1024 |

SetBrakingSettings
​
Sets the Movement Braking Settings of this Character
my_character:SetBrakingSettings(ground_friction?, braking_friction_factor?, braking_walking?, braking_flying?, braking_swimming?, braking_falling?)
Type | Parameter | Default | Description |
---|---|---|---|
float | ground_friction? | 2 | |
float | braking_friction_factor? | 2 | |
integer | braking_walking? | 96 | |
integer | braking_flying? | 3000 | |
integer | braking_swimming? | 10 | |
integer | braking_falling? | 0 |

SetCameraMode
​
Sets the Camera Mode (i.e. Only TPS, FPS or if allow both)
Using FPSOnly CameraMode on AI will lock his body rotation (when using LookAt)
my_character:SetCameraMode(camera_mode)
Type | Parameter | Default | Description |
---|---|---|---|
CameraMode | camera_mode |

SetCameraOffset
​
Sets the Camera Offset (only affects TPS)
my_character:SetCameraOffset(camera_offset)
Type | Parameter | Default | Description |
---|---|---|---|
Vector | camera_offset |

SetCanCrouch
​
Sets if this Character is allowed to Crouch and to Prone
my_character:SetCanCrouch(can_crouch)
Type | Parameter | Default | Description |
---|---|---|---|
boolean | can_crouch |

SetCanAim
​
Sets if this Character is allowed to Aim
my_character:SetCanAim(can_aim)
Type | Parameter | Default | Description |
---|---|---|---|
boolean | can_aim |

SetCanDrop
​
Sets if this Character is allowed to Drop the Picked up item
my_character:SetCanDrop(can_drop)
Type | Parameter | Default | Description |
---|---|---|---|
boolean | can_drop |

SetCanJump
​
Sets if this Character is allowed to Jump
my_character:SetCanJump(can_jump)
Type | Parameter | Default | Description |
---|---|---|---|
boolean | can_jump |

SetCanDive
​
Sets if this Character is allowed to Dive
my_character:SetCanDive(can_dive)
Type | Parameter | Default | Description |
---|---|---|---|
boolean | can_dive |

SetCanSprint
​
Sets if this Character is allowed to Sprint
my_character:SetCanSprint(can_sprint)
Type | Parameter | Default | Description |
---|---|---|---|
boolean | can_sprint |

SetCanGrabProps
​
Sets if this Character is allowed to Grab any Prop
my_character:SetCanGrabProps(can_grab_props)
Type | Parameter | Default | Description |
---|---|---|---|
boolean | can_grab_props |

SetCanPickupPickables
​
Sets if this Character is allowed to Pick up any Pickable (Weapon, Grenade, Melee...)
my_character:SetCanPickupPickables(can_pickup)
Type | Parameter | Default | Description |
---|---|---|---|
boolean | can_pickup |

SetCanPunch
​
Sets if this Character is allowed to Punch
my_character:SetCanPunch(can_punch)
Type | Parameter | Default | Description |
---|---|---|---|
boolean | can_punch |

SetCanDeployParachute
​
Sets if this Character is allowed to deploy the Parachute
my_character:SetCanDeployParachute(can_deploy_parachute)
Type | Parameter | Default | Description |
---|---|---|---|
boolean | can_deploy_parachute |

SetCapsuleSize
​
Sets this Character's Capsule size (will affect Camera location and Character's collision)
my_character:SetCapsuleSize(radius, half_height)

SetDamageMultiplier
​
Changes how much damage this character takes on specific bones
my_character:SetDamageMultiplier(bone_name, multiplier)

SetDeathSound
​
Changes the Death sound when Character dies
my_character:SetDeathSound(sound_asset)
Type | Parameter | Default | Description |
---|---|---|---|
string | sound_asset |

SetFallDamageTaken
​
Set the Fall Damage multiplier taken when falling from High places.
Setting to 0 will make the Character to do not take any damage
my_character:SetFallDamageTaken(damage)
Type | Parameter | Default | Description |
---|---|---|---|
integer | damage | Default is 10 |

SetFlyingMode
​
Sets the Flying Mode
my_character:SetFlyingMode(flying_mode)
Type | Parameter | Default | Description |
---|---|---|---|
boolean | flying_mode |

SetFOVMultiplier
​
Sets the Field of View multiplier
my_character:SetFOVMultiplier(multiplier)
Type | Parameter | Default | Description |
---|---|---|---|
float | multiplier |

SetHighFallingTime
​
Sets time elapsed until automatically transition to HighFalling state (from SmallFalling) in seconds
Set it to -1 to never enter HighFalling time and consequently do not ragdoll when falling
my_character:SetHighFallingTime(time)
Type | Parameter | Default | Description |
---|---|---|---|
float | time | Default is 1 second |

SetGaitMode
​
Sets the Gait Mode
my_character:SetGaitMode(mode)
Type | Parameter | Default | Description |
---|---|---|---|
GaitMode | mode |

SetGravityScale
​
Changes the Gravity Scale of this Character (can be negative)
my_character:SetGravityScale(scale)
Type | Parameter | Default | Description |
---|---|---|---|
float | scale |

SetImpactDamageTaken
​
Set the Impact Damage taken when being roamed by things.
Setting to 0 will make the Character to do not take damage or enter ragdoll mode
my_character:SetImpactDamageTaken(damage)
Type | Parameter | Default | Description |
---|---|---|---|
integer | damage | Default is 10 |

SetRadialDamageToRagdoll
​
Set the minimum radial damage taken (e.g. explosions) to enter in ragdoll mode.
Setting to -1 will make the Character to do not enter ragdoll mode when getting radial damage
my_character:SetRadialDamageToRagdoll(damage)
Type | Parameter | Default | Description |
---|---|---|---|
integer | damage | Default is 50 |

SetFootstepVolumeMultiplier
​
Set the Footstep Volume multiplier
my_character:SetFootstepVolumeMultiplier(volume_multiplier)
Type | Parameter | Default | Description |
---|---|---|---|
float | volume_multiplier |

SetInvulnerable
​
Sets if the Character can receive any damage
my_character:SetInvulnerable(is_invulnerable)
Type | Parameter | Default | Description |
---|---|---|---|
boolean | is_invulnerable |

SetJumpZVelocity
​
Sets the velocity of the jump
my_character:SetJumpZVelocity(velocity)
Type | Parameter | Default | Description |
---|---|---|---|
integer | velocity | Default is 450 |

SetMesh
​
Changes the Character Mesh on the fly
my_character:SetMesh(skeletal_mesh_asset)
Type | Parameter | Default | Description |
---|---|---|---|
SkeletalMesh Reference | skeletal_mesh_asset |
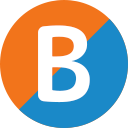
SetMorphTarget
​
Set Morph Target with Name and Value
my_character:SetMorphTarget(name, value)
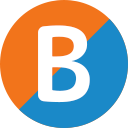
GetMorphTarget
​
Returns the value of a Morph Target
— Returns float (value of the Morph Target).
local ret = my_character:GetMorphTarget(name)
Type | Parameter | Default | Description |
---|---|---|---|
string | name | Morph Target Name |
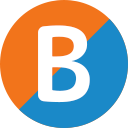
ClearMorphTargets
​
Clear all Morph Target that are set to this Mesh
my_character:ClearMorphTargets()

GetAllMorphTargetNames
​
Returns a table with all morph targets available
— Returns table of string (table with all morph targets available).
local ret = my_character:GetAllMorphTargetNames()
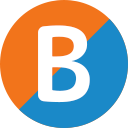
SetPhysicalAnimationSettings
​
Applies the physical animation settings to the body given
my_character:SetPhysicalAnimationSettings(bone, include_self, is_local_simulation, orientation_strength?, angular_velocity_strength?, position_strength?, velocity_strength?, max_linear_force?, max_angular_force?)
Type | Parameter | Default | Description |
---|---|---|---|
string | bone | The body we will be driving | |
boolean | include_self | Whether to modify the given body | |
boolean | is_local_simulation | Whether the drive targets are in world space or local | |
float | orientation_strength? | 0 | The strength used to correct orientation error |
float | angular_velocity_strength? | 0 | The strength used to correct angular velocity error |
float | position_strength? | 0 | The strength used to correct linear position error. Only used for non-local simulation |
float | velocity_strength? | 0 | The strength used to correct linear velocity error. Only used for non-local simulation |
float | max_linear_force? | 0 | The max force used to correct linear errors |
float | max_angular_force? | 0 | The max force used to correct angular errors |
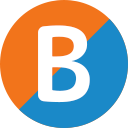
ResetPhysicalAnimationSettings
​
Resets all Physical Animation settings
my_character:ResetPhysicalAnimationSettings()

SetInputEnabled
​
Enables/Disables Character's Input
my_character:SetInputEnabled(is_enabled)
Type | Parameter | Default | Description |
---|---|---|---|
boolean | is_enabled |

SetParachuteTexture
​
Changes the Parachute Texture
my_character:SetParachuteTexture(texture)
Type | Parameter | Default | Description |
---|---|---|---|
Image Path | texture |

SetPainSound
​
Changes the Pain sound when Character takes damage
my_character:SetPainSound(sound_asset)
Type | Parameter | Default | Description |
---|---|---|---|
Sound Reference | sound_asset |

SetPunchDamage
​
Set the Punch Damage this Character will apply on others
my_character:SetPunchDamage(damage)
Type | Parameter | Default | Description |
---|---|---|---|
integer | damage | Default is 15 |

SetRagdollMode
​
Sets Character Ragdoll Mode
my_character:SetRagdollMode(ragdoll_enabled)
Type | Parameter | Default | Description |
---|---|---|---|
boolean | ragdoll_enabled |

SetSimulatePhysics
​
Sets the Character Capsule to simulate physics
my_character:SetSimulatePhysics(simulate_physics)
Type | Parameter | Default | Description |
---|---|---|---|
boolean | simulate_physics |

SetSpeedMultiplier
​
Sets all speed multiplier
my_character:SetSpeedMultiplier(multiplier)
Type | Parameter | Default | Description |
---|---|---|---|
float | multiplier | 1 is normal |

SetStanceMode
​
Sets the Stance Mode
my_character:SetStanceMode(mode)
Type | Parameter | Default | Description |
---|---|---|---|
StanceMode | mode |

SetTeam
​
Sets a Team which will disable damaging same Team Members
my_character:SetTeam(team)
Type | Parameter | Default | Description |
---|---|---|---|
integer | team | 0 is neutral and default |
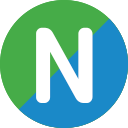
SetViewMode
​
Sets the View Mode
my_character:SetViewMode(view_mode)
Type | Parameter | Default | Description |
---|---|---|---|
ViewMode | view_mode |
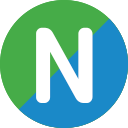
SetWeaponAimMode
​
Sets the Weapon's Aim Mode
my_character:SetWeaponAimMode(aim_mode)
Type | Parameter | Default | Description |
---|---|---|---|
AimMode | aim_mode |

UnGrabProp
​
UnGrabs/Drops the Prop the Character is holding
my_character:UnGrabProp()
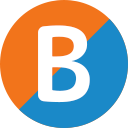
IsInRagdollMode
​
Gets if Character is in ragdoll mode
— Returns boolean.
local ret = my_character:IsInRagdollMode()
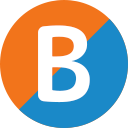
IsInvulnerable
​
Gets if is invulnerable
— Returns boolean.
local ret = my_character:IsInvulnerable()
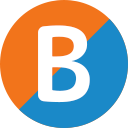
IsInputEnabled
​
Gets if has input enabled
— Returns boolean.
local ret = my_character:IsInputEnabled()
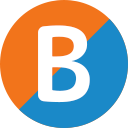
GetCameraMode
​
Gets the camera mode
— Returns CameraMode.
local ret = my_character:GetCameraMode()
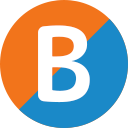
GetCanDrop
​
Gets if can drop
— Returns boolean.
local ret = my_character:GetCanDrop()
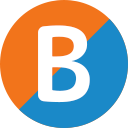
GetCanPunch
​
Gets if can punch
— Returns boolean.
local ret = my_character:GetCanPunch()
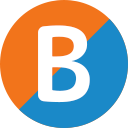
GetCanAim
​
Gets if can aim
— Returns boolean.
local ret = my_character:GetCanAim()
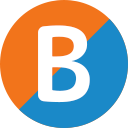
GetCanCrouch
​
Gets if can crouch
— Returns boolean.
local ret = my_character:GetCanCrouch()
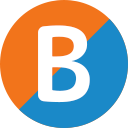
GetCanSprint
​
Gets if can sprint
— Returns boolean.
local ret = my_character:GetCanSprint()
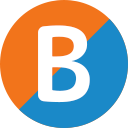
GetCanGrabProps
​
Gets if can grab props
— Returns boolean.
local ret = my_character:GetCanGrabProps()
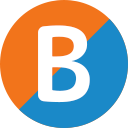
GetCanPickupPickables
​
Gets if can pickup Pickables (Weapons, Melee, Grenade...)
— Returns boolean.
local ret = my_character:GetCanPickupPickables()
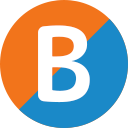
GetCapsuleSize
​
Gets the Capsule Size
— Returns table (with this format).
local ret = my_character:GetCapsuleSize()

GetBoneTransform
​
Gets a Bone Transform in world space given a bone name
— Returns table (with this format).
local ret = my_character:GetBoneTransform(bone_name)
Type | Parameter | Default | Description |
---|---|---|---|
string | bone_name |
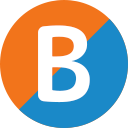
GetControlRotation
​
Gets the Control Rotation
— Returns Rotator.
local ret = my_character:GetControlRotation()
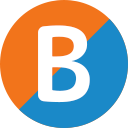
GetDamageMultiplier
​
Gets the Damage Multiplier of a bone
— Returns float (the damage multiplier of the bone).
local ret = my_character:GetDamageMultiplier(bone_name)
Type | Parameter | Default | Description |
---|---|---|---|
string | bone_name |
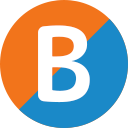
GetFallDamageTaken
​
Gets the Fall Damage
— Returns integer.
local ret = my_character:GetFallDamageTaken()
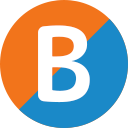
GetFallingMode
​
Gets the FallingMode
— Returns FallingMode.
local ret = my_character:GetFallingMode()
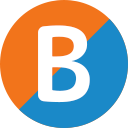
GetFlyingMode
​
Gets if it's in Flying mode
— Returns boolean.
local ret = my_character:GetFlyingMode()
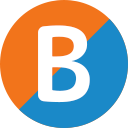
GetGaitMode
​
Gets the GaitMode
— Returns GaitMode.
local ret = my_character:GetGaitMode()
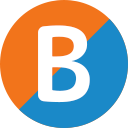
GetGrabbedProp
​
Gets the Grabbed Prop
local ret = my_character:GetGrabbedProp()
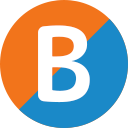
GetGravityScale
​
Gets the gravity scale
— Returns float.
local ret = my_character:GetGravityScale()
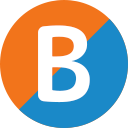
GetImpactDamageTaken
​
Gets the impact damage taken
— Returns integer.
local ret = my_character:GetImpactDamageTaken()
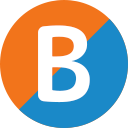
GetJumpZVelocity
​
Gets the Jump Z Velocity
— Returns integer.
local ret = my_character:GetJumpZVelocity()
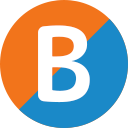
GetMesh
​
Gets the Skeletal Mesh Asset
— Returns SkeletalMesh Reference.
local ret = my_character:GetMesh()
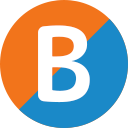
GetMovingTo
​
Gets the Moving To location
— Returns Vector (the moving to location or Vector(0, 0, 0) if not moving).
local ret = my_character:GetMovingTo()
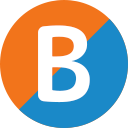
GetPicked
​
Gets the Pickable if picking up
— Returns Base Pickable or nil.
local ret = my_character:GetPicked()
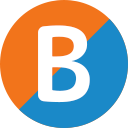
GetPlayer
​
Gets the possessing Player
local ret = my_character:GetPlayer()
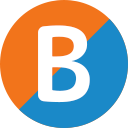
GetPunchDamage
​
Gets the punch damage
— Returns integer.
local ret = my_character:GetPunchDamage()
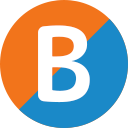
GetSpeedMultiplier
​
Gets the speed multiplier
— Returns float.
local ret = my_character:GetSpeedMultiplier()
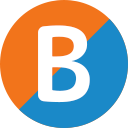
GetStanceMode
​
Gets the Stance Mode
— Returns StanceMode.
local ret = my_character:GetStanceMode()
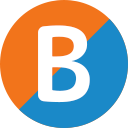
GetSwimmingMode
​
Gets the Swimming Mode
— Returns SwimmingMode.
local ret = my_character:GetSwimmingMode()
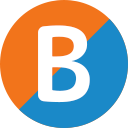
GetTeam
​
Gets the Team
— Returns integer.
local ret = my_character:GetTeam()
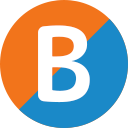
GetVehicle
​
Gets the entered Vehicle
local ret = my_character:GetVehicle()
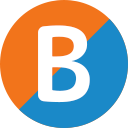
GetViewMode
​
Gets the View Mode
— Returns ViewMode.
local ret = my_character:GetViewMode()
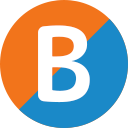
GetWeaponAimMode
​
Gets the Weapon Aim Mode
— Returns AimMode.
local ret = my_character:GetWeaponAimMode()
Events​
Inherited Entity Events
Name | Description | |
---|---|---|
![]() | Spawn | Triggered when an Entity is spawned/created |
![]() | Destroy | Triggered when an Entity is destroyed |
![]() | ValueChange | Triggered when an Entity has a value changed with :SetValue() |
![]() | ClassRegister | Triggered when a new Class is registered with the Inheriting System |
Inherited Actor Events
Name | Description | |
---|---|---|
![]() | Spawn | Triggered when an Entity is spawned/created |
![]() | Destroy | Triggered when an Entity is destroyed |
![]() | ValueChange | Triggered when an Entity has a value changed with :SetValue() |
![]() | ClassRegister | Triggered when a new Class is registered with the Inheriting System |
Inherited Damageable Events
Name | Description | |
---|---|---|
![]() | Spawn | Triggered when an Entity is spawned/created |
![]() | Destroy | Triggered when an Entity is destroyed |
![]() | ValueChange | Triggered when an Entity has a value changed with :SetValue() |
![]() | ClassRegister | Triggered when a new Class is registered with the Inheriting System |
Name | Description | |
---|---|---|
AnimationBeginNotify | When an Animation Montage Notify begins | |
AnimationEndNotify | When an Animation Montage Notify ends | |
![]() | Attack | Triggered when the Character effectively attacks with a Melee |
![]() | Drop | When Character drops the currently picked up Pickable |
![]() | EnterVehicle | When Character enters a vehicle |
AttemptEnterVehicle | Triggered when a Character attempts to enter a vehicle | |
![]() | FallingModeChange | Called when FallingMode changes |
![]() | Fire | When Character fires a Weapon |
![]() | GaitModeChange | Called when GaitMode changes |
![]() | GrabProp | When Character grabs up a Prop |
![]() | Highlight | When Character highlights/looks at a Prop or a Pickable |
Interact | Triggered when a Character interacts with a Prop or Pickable | |
![]() | LeaveVehicle | When Character leaves a vehicle |
AttemptLeaveVehicle | Triggered when this Character attempts to leave a vehicle | |
![]() | MoveComplete | Called when AI reaches it's destination, or when it fails |
![]() | PickUp | When Character picks up anything |
![]() | Possess | When Character is possessed |
![]() | Punch | When Character punches |
![]() | RagdollModeChange | When Character enters or leaves ragdoll |
![]() | AttemptReload | Triggered when this Character attempts to reload |
![]() | Reload | When Character reloads a weapon |
![]() | StanceModeChange | Called when StanceMode changes |
![]() | SwimmingModeChange | Called when Swimming Mode changes |
![]() | UnGrabProp | When Character drops a Prop |
![]() | UnPossess | When Character is unpossessed |
![]() | ViewModeChange | When Character changes it's View Mode |
![]() | WeaponAimModeChange | Called when Weapon Aim Mode changes |
![]() | PullUse | Triggered when a Character presses the use button for a Pickable (i.e. clicks left mouse button with this equipped) |
![]() | ReleaseUse | Triggered when a Character releases the use button for a Pickable (i.e. releases left mouse button with this equipped) |

AnimationBeginNotify
​
When an Animation Montage Notify begins
Character.Subscribe("AnimationBeginNotify", function(self, notify_name)
-- AnimationBeginNotify was called
end)

AnimationEndNotify
​
When an Animation Montage Notify ends
Character.Subscribe("AnimationEndNotify", function(self, notify_name)
-- AnimationEndNotify was called
end)
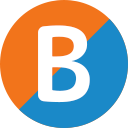
Attack
​
Triggered when the Character effectively attacks with a Melee
Character.Subscribe("Attack", function(self, melee)
-- Attack was called
end)
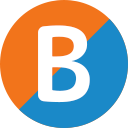
Drop
​
When Character drops the currently picked up Pickable
Character.Subscribe("Drop", function(self, object, triggered_by_player)
-- Drop was called
end)
Type | Argument | Description |
---|---|---|
Character | self | |
Base Pickable | object | |
boolean | triggered_by_player |
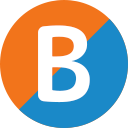
EnterVehicle
​
When Character enters a vehicle
Character.Subscribe("EnterVehicle", function(self, vehicle, seat_index)
-- EnterVehicle was called
end)

AttemptEnterVehicle
​
Triggered when a Character attempts to enter a vehicle
Returnfalse
to prevent it
Character.Subscribe("AttemptEnterVehicle", function(self, vehicle, seat_index)
-- AttemptEnterVehicle was called
end)
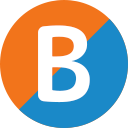
FallingModeChange
​
Called when FallingMode changes
Character.Subscribe("FallingModeChange", function(self, old_state, new_state)
-- FallingModeChange was called
end)
Type | Argument | Description |
---|---|---|
Character | self | |
FallingMode | old_state | |
FallingMode | new_state |
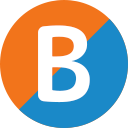
Fire
​
When Character fires a Weapon
Character.Subscribe("Fire", function(self, weapon)
-- Fire was called
end)
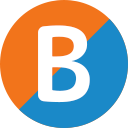
GaitModeChange
​
Called when GaitMode changes
Character.Subscribe("GaitModeChange", function(self, old_state, new_state)
-- GaitModeChange was called
end)
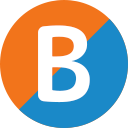
GrabProp
​
When Character grabs up a Prop
Character.Subscribe("GrabProp", function(self, prop)
-- GrabProp was called
end)
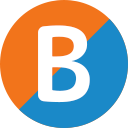
Highlight
​
When Character highlights/looks at a Prop or a Pickable
Character.Subscribe("Highlight", function(self, is_highlighted, object)
-- Highlight was called
end)
Type | Argument | Description |
---|---|---|
Character | self | |
boolean | is_highlighted | Whether the object is being highlighted or not |
Prop or Base Pickable | object |

Interact
​
Triggered when a Character interacts with a Prop or Pickable
Returnfalse
to prevent it
Character.Subscribe("Interact", function(self, object)
-- Interact was called
end)
Type | Argument | Description |
---|---|---|
Character | self | |
Prop or Base Pickable | object |
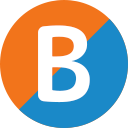
LeaveVehicle
​
When Character leaves a vehicle
Character.Subscribe("LeaveVehicle", function(self, vehicle)
-- LeaveVehicle was called
end)

AttemptLeaveVehicle
​
Triggered when this Character attempts to leave a vehicle
Returnfalse
to prevent it
Character.Subscribe("AttemptLeaveVehicle", function(self, vehicle)
-- AttemptLeaveVehicle was called
end)
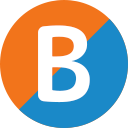
MoveComplete
​
Called when AI reaches it's destination, or when it fails
Character.Subscribe("MoveComplete", function(self, succeeded)
-- MoveComplete was called
end)
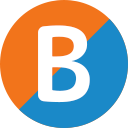
PickUp
​
When Character picks up anything
Character.Subscribe("PickUp", function(self, object)
-- PickUp was called
end)
Type | Argument | Description |
---|---|---|
Character | self | |
Base Pickable | object |
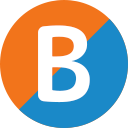
Possess
​
When Character is possessed
Character.Subscribe("Possess", function(self, possesser)
-- Possess was called
end)
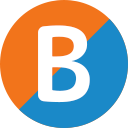
Punch
​
When Character punches
Character.Subscribe("Punch", function(self)
-- Punch was called
end)
Type | Argument | Description |
---|---|---|
Character | self |
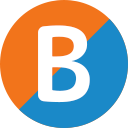
RagdollModeChange
​
When Character enters or leaves ragdoll
Character.Subscribe("RagdollModeChange", function(self, old_state, new_state)
-- RagdollModeChange was called
end)
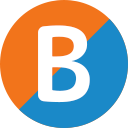
AttemptReload
​
Triggered when this Character attempts to reload
Returnfalse
to prevent it
Character.Subscribe("AttemptReload", function(self, weapon)
-- AttemptReload was called
end)
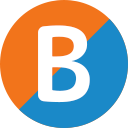
Reload
​
When Character reloads a weapon
Character.Subscribe("Reload", function(self, weapon, ammo_to_reload)
-- Reload was called
end)
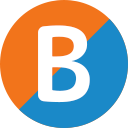
StanceModeChange
​
Called when StanceMode changes
Character.Subscribe("StanceModeChange", function(self, old_state, new_state)
-- StanceModeChange was called
end)
Type | Argument | Description |
---|---|---|
Character | self | |
StanceMode | old_state | |
StanceMode | new_state |
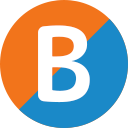
SwimmingModeChange
​
Called when Swimming Mode changes
Character.Subscribe("SwimmingModeChange", function(self, old_state, new_state)
-- SwimmingModeChange was called
end)
Type | Argument | Description |
---|---|---|
Character | self | |
SwimmingMode | old_state | |
SwimmingMode | new_state |
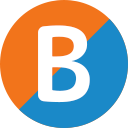
UnGrabProp
​
When Character drops a Prop
Character.Subscribe("UnGrabProp", function(self, prop)
-- UnGrabProp was called
end)
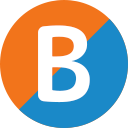
UnPossess
​
When Character is unpossessed
Character.Subscribe("UnPossess", function(self, old_possesser)
-- UnPossess was called
end)
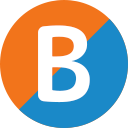
ViewModeChange
​
When Character changes it's View Mode
Character.Subscribe("ViewModeChange", function(self, old_state, new_state)
-- ViewModeChange was called
end)
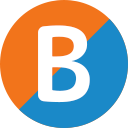
WeaponAimModeChange
​
Called when Weapon Aim Mode changes
Character.Subscribe("WeaponAimModeChange", function(self, old_state, new_state)
-- WeaponAimModeChange was called
end)
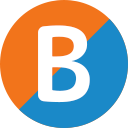
PullUse
​
Triggered when a Character presses the use button for a Pickable (i.e. clicks left mouse button with this equipped)
Character.Subscribe("PullUse", function(self, pickable)
-- PullUse was called
end)
Type | Argument | Description |
---|---|---|
Character | self | The Character that used it |
Base Pickable | pickable | The Pickable which has just been used |
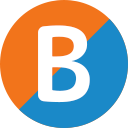
ReleaseUse
​
Triggered when a Character releases the use button for a Pickable (i.e. releases left mouse button with this equipped)
Character.Subscribe("ReleaseUse", function(self, pickable)
-- ReleaseUse was called
end)
Type | Argument | Description |
---|---|---|
Character | self | The Character that stopped using it |
Base Pickable | pickable | The Pickable which has just stopped being used |
HELIX Character Meshes list​
Head​
helix::SK_Female_Head
helix::SK_Male_Head
Beard​
helix::SK_Beard_01
helix::SK_Beard_02
Chest​
helix::SK_Male_Chest
helix::SK_Hoodie_Logo_Man
helix::SK_Man_Outwear_01
helix::SK_Man_Outwear_02
helix::SK_Man_Outwear_03
helix::SK_Man_Outwear_05
helix::SK_Man_Outwear_06
helix::SK_Man_Outwear_07
helix::SK_Man_T_Shirts_01
helix::SK_Man_T_Shirts_02
helix::SK_Man_T_Shirts_03
helix::SK_Man_T_Shirts_04
helix::SK_Wife_Beater
Feet​
helix::SK_Male_Feet
helix::SK_Man_Boots_02
helix::SK_Man_Boots_04
helix::SK_Man_Boots_05
helix::SK_Man_Boots_06
helix::SK_Man_Boots_07
helix::SK_Man_Boots_08
helix::SK_Man_Boots_10
helix::SK_Man_Boots_11
helix::SK_Man_Boots_12
helix::SK_Man_Boots_13
helix::SK_Man_Boots_14
helix::SK_Man_Boots_15
helix::SK_Man_Boots_16
helix::SK_Man_Boots_17
helix::SK_Man_Boots_18
helix::SK_Man_Boots_19
helix::SK_Man_Boots_20
helix::SK_Man_Boots_21
helix::SK_Man_Boots_23
helix::SK_Man_Boots_24
helix::SK_Man_Boots_25
Hands​
helix::SK_Male_Hands
Legs​
helix::SK_Male_Legs
helix::SK_Man_Pants_01
helix::SK_Man_Pants_02
helix::SK_Man_Pants_03
helix::SK_Man_Pants_05
helix::SK_Man_Pants_06
helix::SK_Man_Pants_08
helix::SK_Man_Pants_09
helix::SK_Man_Pants_10
helix::SK_Man_Pants_12
helix::SK_Man_Pants_13
helix::SK_Man_Pants_14
helix::SK_Man_Pants_15
helix::SK_Man_Pants_Short_01
helix::SK_Man_Pants_Short_02
helix::SK_Man_Pants_Short_03
helix::SK_Man_Pants_Short_04
Etc​
helix::SK_Eyebrows_01
helix::SK_Eyebrows_02
helix::SK_Eyelashes
helix::SK_Eyelashes_Shape_01
helix::SK_Eyelashes_Shape_02
helix::SK_Nails_01
helix::SK_Nails_02
helix::SK_Invisible
Delivery​
helix::SK_Delivery_Shoes
helix::SK_Delivery_Top
helix::SK_Delivery_Lower
Police​
helix::SK_Police_Lower
helix::SK_Police_Shoes
helix::SK_Police_Top
helix::SK_Police_Hat
Full Body​
helix::SK_Female_Body
helix::SK_Male
Character's Skeleton Bone Names​
Ugly list I know.
root
pelvis
spine_01
spine_02
spine_03
clavicle_l
upperarm_l
lowerarm_l
hand_l
index_01_l
index_02_l
index_03_l
middle_01_l
middle_02_l
middle_03_l
pinky_01_l
pinky_02_l
pinky_03_l
ring_01_l
ring_02_l
ring_03_l
thumb_01_l
thumb_02_l
thumb_03_l
weapon_l
clavicle_r
upperarm_r
lowerarm_r
hand_r
index_01_r
index_02_r
index_03_r
middle_01_r
middle_02_r
middle_03_r
pinky_01_r
pinky_02_r
pinky_03_r
ring_01_r
ring_02_r
ring_03_r
thumb_01_r
thumb_02_r
thumb_03_r
weapon_r
neck_01
head
lefteye
righteye
leftlidup
leftlidlow
rightlidup
rightlitlow
thigh_l
calf_l
foot_l
ball_l
thigh_r
calf_r
foot_r
ball_r